JavaScript SDK – Overview
Introduction
This page gives an overview of the important methods provided by the Chat SDK that will be required for developing your Web Client. For the complete list of methods and types exposed by SDK, refer to the documentation provided inside the Chat SDK package, more details here.
Usage
Before using the Chat SDK please ensure that the chat integration provisioning requirements are fulfilled. To use the Chat SDK you must provide the integrationId
, a valid JWT Token, and an appkey
during the initialization. Refer to this page on on how to provision the chat integration, get the integrationId
and appkey
, and generate a valid JWT Token.
The Chat SDK can imported in a webpage using the HTML <script>
tag.
<script src="path/to/avaya-ccaas-chat-sdk.js" defer></script>
Initialization
The Chat SDK must be initialized before any further actions can be performed. The Chat SDK is initialized using the AvayaCCaaSChatSDK.init()
method which takes in the required information along with optional configuration(s).
The AvayaCCaaSChatSDK.init()
method takes two arguments, the initialization parameters and any optional configuration values.
Once the initialization is complete SDK resolves the promise with object of type UserContext
which contains details about the session and ongoing chats across all the sessions of the associated User.
Call signature example of AvayaCCaaSChatSDK.init()
:
const configs = {
reconnectionTimeout: 120,
idleTimeout: 300,
idleShutdownGraceTimeout: 30,
logLevel: "DEBUG"
}
let userContext = await AvayaCCaaSChatSDK.init({
hostURL: "<CCaaS Host URL>",
integrationId: "<Integration Id>",
token: "jwt.header.payload",
appkey: "<Application Key>",
sessionParameters: {
"model": "ABC-123"
}
}, configs);
Waiting for initialization to complete
Since the initialization is an asynchronous process, the Client must wait until the SDK is ready to be used before performing any further operations. This can be done by waiting for the result of the Promise returned by the AvayaCCaaSChatSDK.init()
method.
Chat SDK also raises the INITIALIZED
event after initialization is complete. A Client can subscribe to this event using the AvayaCCaaSChatSDK.on()
method and perform additional operations in the event handler function passed as a parameter to this method. Once the INITIALIZED
event has occurred, this event handler function will be called, and the same object (the UserContext
) which is available in the resolved promise returned by AvayaCCaaSChatSDK.init()
method will also be passed to this event handler function.
Info
In order to handle the
INITIALIZED
event, the client must subscribe to this event before callingAvayaCCaaSChatSDK.init()
. It is also recommended to subscribe to all the events before making the initialization attempt. Refer to Event Handling section to understand how to handle the events raised by the SDK.
Example:
// Attach the handler for 'INITIALIZED' event before making the AvayaCCaaSChatSDK.init() call
AvayaCCaaSChatSDK.on('INITIALIZED', (userContext) => {
// Code to run after initialization
});
// Init parameters are not shown in this example for brevity.
AvayaCCaaSChatSDK.init(...).then((userContext) => {
// Code to run after initialization
});
This can also be achieved using the await
syntax.
Example:
// Attach the handler for 'INITIALIZED' event before making the AvayaCCaaSChatSDK.init() call
AvayaCCaaSChatSDK.on('INITIALIZED', (userContext) => {
// Code to run after initialization
});
// Init parameters are not shown in this example for brevity.
let userContext = await AvayaCCaaSChatSDK.init(...);
Authentication
Chat SDK requires a valid JWT and appkey
to connect to Avaya Experience Platform™ Digital. The JWT and appkey
must be passed as a part of initialization parameters to the init()
method.
Since the validity of the JWT is limited, it must be refreshed periodically. The Chat SDK provides two events for this exact purpose and the refreshing logic can be written in their handlers.
Detailed information about the appkey
, JWT and how it can be retrieved, can be found here.
JWT Lifecycle Events
Chat SDK will emit the TOKEN_EXPIRY_REMINDER
event precisely three minutes before the JWT expires. This event is raised so that the Client can contact the backend web-application to retrieve a new JWT and set it in the SDK before the existing JWT expires.
Warning
If the Client fails to set a new JWT before the existing JWT expires,
TOKEN_EXPIRED
event is raised and the Chat SDK remains in a disconnected state until a valid JWT is set.
Once a JWT is re-generated it can be set using the Chat SDK’s AvayaCCaaSChatSDK.setJwtToken()
method.
function refreshJWT(details) {
// Fetch the new JWT token from your backend web application.
// ...
AvayaCCaaSChatSDK.setJwtToken(token);
}
AvayaCCaaSChatSDK.on('TOKEN_EXPIRY_REMINDER', refreshJWT);
AvayaCCaaSChatSDK.on('TOKEN_EXPIRED', refreshJWT);
Event Handling
Whenever an event occurs on the Avaya Experience Platform™ Digital for User's engagements joined through the SDK session, or there are state changes within the SDK, events are emitted by SDK to notify the Client so that it can update its UI accordingly. The Client can subscribe to these events using the Chat SDK’s AvayaCCaaSChatSDK.on()
method which takes two arguments namely the event name and its associated handler.
AvayaCCaaSChatSDK.on('MESSAGE_ARRIVED', (message) => {
// Show message on UI.
});
Similarly, the Client can unsubscribe to an individual event or all the events using the Chat SDK’s AvayaCCaaSChatSDK.off()
method.
// Remove event handler for specific event.
AvayaCCaaSChatSDK.off('MESSAGE_ARRIVED');
// Remove event handlers for all events.
AvayaCCaaSChatSDK.off();
Working with chats
Chat SDK provides an Engagement
object for each ongoing active chats across all the sessions associated to the User. All operations that can be performed on the chat like joining it, sending messages etc. can be performed using this Engagement
object.
Info
The Chat SDK must be initialized before any ongoing chat engagements can be accessed, or a new chat can be started.
Starting a new chat
To start a new chat, a new engagement must be created using AvayaCCaaSChatSDK.createEngagement()
. You can pass additional information about the engagement in the optional parameter engagementParameters
to this method. This information can be used for 'Context Data Matching' if 'Routing Configuration Settings' of the Chat Integration is configured appropriately by your Account Administrator.
const engagement = await AvayaCCaaSChatSDK.createEngagement({
"availableBalance" : "100"
});
List all ongoing chats
List of all ongoing chat engagements across all the sessions of the User can be obtained by using AvayaCCaaSChatSDK.getEngagements()
.
const engagements = await AvayaCCaaSChatSDK.getEngagements();
Getting specific chat details
The Client can get the information for a specific engagement calling AvayaCCaaSChatSDK.getEngagementById()
and passing the id for that engagement.
const engagement = AvayaCCaaSChatSDK.getEngagementById("<engagement id>");
Joining an ongoing chat
In case the user wants to continue to chat on an ongoing engagement that might have been started on a different session, the Engagement
object's join()
method should be called.
const engagement = AvayaCCaaSChatSDK.getEngagementById("<engagement id>");
await engagement.join();
Disconnecting the chat
The Engagement object's disconnect()
method allows the User to disconnect from that Engagement.
Info
Engagement must be active before it can be disconnected.
await engagement.disconnect();
Getting Participant details
The Engagement object's participants
accessor and getParticipantById()
method provides list of all Participants
that are currently part of the chat.
const engagement = AvayaCCaaSChatSDK.getEngagementById("<engagement id>");
const participants = engagement.getParticipants();
const participant = engagement.getParticipantById("<participant id>");
Chat Engagement related Events
Updates related to all the active engagements are delivered via the following Events:
Event | Description |
---|---|
ENGAGEMENT_ACTIVE | Emitted when the User has been successfully added as a Participant into the chat |
PARTICIPANT_ADDED | Emitted when any new Participant is added into the chat |
PARTICIPANT_DISCONNECTED | Emitted when a participant is disconnected from the chat |
ENGAGEMENT_TERMINATED | Emitted when the chat has ended for the User |
ENGAGEMENT_ERROR | Emitted when there is an error regarding the chat |
MESSAGE_ARRIVED | Emitted when a new message has arrived. |
MESSAGE_DELIVERED | Emitted when the message sent by the user is delivered. |
The Client can show appropriate notifications on the UI by subscribing to these events.
List of all events are available under the documentation provided inside the Chat SDK package, more details here.
Messaging
The Client can send and receive messages in a Chat Engagement using the Chat SDK.
Sending a message
A message can be sent using the Engagement object's sendMessage()
method. The User's message can be passed as a string to this method.
Once sending is complete, the promise returned by sendMessage()
will be resolved with the a Message
object corresponding to the message that was sent. This object contains unique messageId
of this message and other details.
const message = await engagement.sendMessage("Hello");
console.log(message.messageId);
Message Delivery
The Client must subscribe to the MESSAGE_DELIVERED
event to be notified when the messages that were sent by the User are delivered.
AvayaCCaaSChatSDK.on('MESSAGE_DELIVERED', (message) = {
// Show tick on UI.
});
Receiving Messages
The Client must subscribe to the MESSAGE_ARRIVED
event to receive messages from other Participants in the chat. The MessageArrived
object will be passed to the handler subscribing to this event.
AvayaCCaaSChatSDK.on('MESSAGE_ARRIVED', (message) = {
// Show new message on UI.
});
Fetching all messages of an ongoing chat
All the messages that have been exchanged as part of the chat can be fetched using the Engagement object’s getMessages()
method. This method is helpful specially when the User or Client joins an ongoing Engagement that might have been started from a different session or in situations of a browser refresh.
This method returns an Iterator object for iterating over the pages of messages. It takes the page size as argument which defaults to 10 if not provided.
The iterator object can then be used for fetching all the messages exchanged in that Engagement, right from the first message to the last message exchanged before the point of time it was returned by the method.
Warning
Any new messages which are exchanged after the PageIterator's creation are not present in that instance of PageIterator. Subscribe to the
MESSAGE_ARRIVED
event to receive any new messages. Do not call the Engagement object'sgetMessages()
method in a loop.
function showMessagesOnUI(messages) {
// Logic to show messages on UI.
// ...
}
const iterator = await engagement.getMessages(15);
showMessagesOnUI(iterator.items);
const loadMore = document.getElementById('load-more-button');
loadMore.onclick = function () {
if (iterator.hasPrevious()) {
await iterator.previous();
showMessagesOnUI(iterator.items);
}
}
User activity and Timeouts
As Chat SDK is session based, the Chat SDK provides mechanism to automatically clear up the session if the User's session has not been active for configured amount of time. The following sections explain what is considered as User Activity along with various timers and events that support this feature.
User Activity
When one of the following methods are invoked by the Client, the SDK automatically considers that the User is actively using the SDK session:
- AvayaCCaaSChatSDK.createEngagement()
- Engagement.disconnect()
- Engagement.sendMessage()
- Engagement.join()
Timeouts
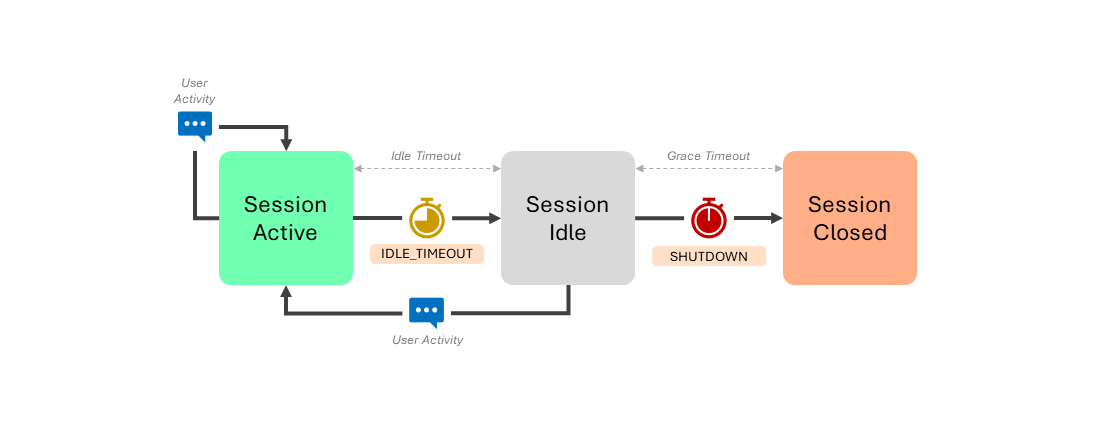
Timeouts
Chat SDK runs two user inactivity timers.
The first timer is the idle timer which is started right after the session is created. Any activity from the User or Client (mentioned above) resets this timer. This timer expires when there are no activities for the configured duration. Once this timer expires the SDK will emit the IDLE_TIMEOUT_EXPIRED
event. The Client can show an appropriate message on the UI, warning the User about inactivity, by handling this event.
The second timer is idle shutdown grace timer which runs after the idle timer has expired. This timer provides additional grace period for User or the Client to extend the session. After this timer expires, the session is terminated automatically and the SDK will raise the SHUTDOWN
event. If the Client wants to continue it must be reinitialize the SDK to continue.
Both the timeout values can be configured during the initialization by providing their values in the Config
object (second parameter of AvayaCCaaSChatSDK.init()
method). The idleTimeout must be between 300 seconds and 3300 seconds (defaults to 300 seconds) while the idleShutdownGraceTimeout value must be between 30 seconds to 120 seconds (defaults to 30 seconds).
Example:
// First parameters is not shown in this example for brevity.
AvayaCCaaSChatSDK.init(sdkInitParams, {
idleTimeout: 900,
idleShutdownGraceTimeout: 120
});
// ...
function warnUser(message) {
// Show warning on UI.
}
AvayaCCaaSChatSDK.on('IDLE_TIMEOUT_EXPIRED', (eventPayload) => {
warnUser(`The session will expire in ${eventPayload.gracePeriod}`);
});
Extending the Session
SDK provides the AvayaCCaaSChatSDK.resetIdleTimeout()
method for the Client to reset the idle timer and idle shutdown grace timer timers. Unlike user activities
which are actions on engagements, AvayaCCaaSChatSDK.resetIdleTimeout()
only impacts the timers. This method helps the Client to extend the session in scenarios where no action is being taken by the User on engagements but the Client, based on its events from UI is aware that the User is active.
Info
The Chat SDK doesn't close any of the ongoing chats when the Session is terminated due to inactivity as the User might be engaged on those chats through another session.
function showWarningBox(eventPayload) {
const continueChatButton = document.getElementById('inactivity-warning-continue-chat');
continueChatButton.onclick = () => {
AvayaCCaaSChatSDK.resetIdleTimeout();
}
// ...
setTimeout(hideWarningBox, eventPayload.gracePeriod);
}
function hideWarningBox() {
// ...
}
AvayaCCaaSChatSDK.on('IDLE_TIMEOUT', showWarningBox);
Network
During the session, the state of SDK’s connection with Avaya Experience Platform™ can change. In all the cases the network state changes are notified in the form of events. The Client can subscribe to these events for handling the changes in network.
State Name | Description |
---|---|
EVENT_STREAM_CONNECTING | This event is raised when the SDK tries to connect with the event stream. |
EVENT_STREAM_CONNECTED | This event is raised when the SDK's connection attempt was successful, and the connection with Avaya Experience Platform™ is established. |
EVENT_STREAM_FAILED | This event is raised when the event stream breaks/fails due to some reason. Details like the reason for failure as well as next retry attempt is provided in the event. |
EVENT_STREAM_CLOSED | This event is raised when the SDK disconnects itself from the event stream. |
AvayaCCaaSChatSDK.on('EVENT_STREAM_CONNECTING', (eventPayload) => {
// Show connecting on UI.
});
AvayaCCaaSChatSDK.on('EVENT_STREAM_CONNECTED', (eventPayload) => {
// Show connected on UI.
});
AvayaCCaaSChatSDK.on('EVENT_STREAM_FAILED', (eventPayload) => {
// Show network disconnected on UI.
});
AvayaCCaaSChatSDK.on('EVENT_STREAM_CLOSED', (eventPayload) => {
// Show connection closed on UI.
});
After disconnection, the SDK will try to reconnect with Avaya Experience Platform™ until the reconnection timeout expires. This reconnection timeout can be configured during initialization and defaults 120 seconds. Minimum value is 120 seconds and maximum is 300 seconds.
// First parameters is not shown in this example for brevity.
AvayaCCaaSChatSDK.init(sdkInitParams, {
reconnectionTimeout: 300
});
Shutdown
Current user's session can be ended using the SDK's AvayaCCaaSChatSDK.shutdown()
method. This will end the session and cleanup all the data corresponding to that session from SDK. User will not lose any ongoing chats because of a session shutdown.
The Chat SDK also raises the SHUTDOWN
event after the shutdown is complete.
Warning
Once the SDK is shutdown the Engagement objects are also removed, hence the Client must re-initialize the SDK incase it wants to start again.
AvayaCCaaSChatSDK.on('SHUTDOWN', (eventPayload) => {
// Do UI cleanup after shutdown.
});
await AvayaCCaaSChatSDK.shutdown();
// OR
AvayaCCaaSChatSDK.shutdown().then((data) => {
// Do UI cleanup after shutdown.
});
// OR
await AvayaCCaaSChatSDK.shutdown();
// Do UI cleanup after shutdown.
Updated 8 months ago